To provide a widget for selecting a date, use the DatePicker
widget, which allows the user to select the month, day, and year, in a familiar interface.
In this tutorial, you'll create a DatePickerDialog
, which presents the
date picker in a floating dialog box at the press of a button. When the date is set by
the user, a TextView
will update with the new date.
- Start a new project named HelloDatePicker.
- Open the
res/layout/main.xml
file and insert the following:<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="wrap_content" android:layout_height="wrap_content" android:orientation="vertical"> <TextView android:id="@+id/dateDisplay" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text=""/> <Button android:id="@+id/pickDate" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Change the date"/> </LinearLayout>
This creates a basic
LinearLayout
with aTextView
that will display the date and aButton
that will open theDatePickerDialog
. - Open
HelloDatePicker.java
and add the following members to the class:private TextView mDateDisplay; private Button mPickDate; private int mYear; private int mMonth; private int mDay; static final int DATE_DIALOG_ID = 0;
The first group of members define variables for the layout
View
s and the date items. TheDATE_DIALOG_ID
is a static integer that uniquely identifies theDialog
that will display the date picker. - Now add the following code for the
onCreate()
method:protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); // capture our View elements mDateDisplay = (TextView) findViewById(R.id.dateDisplay); mPickDate = (Button) findViewById(R.id.pickDate); // add a click listener to the button mPickDate.setOnClickListener(new View.OnClickListener() { public void onClick(View v) { showDialog(DATE_DIALOG_ID); } }); // get the current date final Calendar c = Calendar.getInstance(); mYear = c.get(Calendar.YEAR); mMonth = c.get(Calendar.MONTH); mDay = c.get(Calendar.DAY_OF_MONTH); // display the current date (this method is below) updateDisplay(); }
First, the content is set to the
main.xml
layout. Then theTextView
andButton
elements are captured from the layout withfindViewById(int)
. A newView.OnClickListener
is created for theButton
, so that when it is clicked, it will callshowDialog(int)
, passing the unique integer ID for the date picker dialog. UsingshowDialog(int)
allows theActivity
to manage the life-cycle of the dialog and will call theonCreateDialog(int)
callback method to request theDialog
that should be displayed (which you'll define later). After the on-click listener is set, a newCalendar
is created and the current year, month and day are acquired. Finally, the privateupdateDisplay()
method is called in order to fill theTextView
with the current date. - Add the
updateDisplay()
method:// updates the date in the TextView private void updateDisplay() { mDateDisplay.setText( new StringBuilder() // Month is 0 based so add 1 .append(mMonth + 1).append("-") .append(mDay).append("-") .append(mYear).append(" ")); }
This method uses the member date values declared for the class to write the date to the layout's
TextView
,mDateDisplay
, which was also declared and initialized above. - Initialize a new
DatePickerDialog.OnDateSetListener
as a member of theHelloDatePicker
class:// the callback received when the user "sets" the date in the dialog private DatePickerDialog.OnDateSetListener mDateSetListener = new DatePickerDialog.OnDateSetListener() { public void onDateSet(DatePicker view, int year, int monthOfYear, int dayOfMonth) { mYear = year; mMonth = monthOfYear; mDay = dayOfMonth; updateDisplay(); } };
The
DatePickerDialog.OnDateSetListener
listens for when the user has set the date (by clicking the "Set" button). At that time, theonDateSet()
callback method is called, which is defined to update themYear
,mMonth
, andmDay
member fields with the new date then call the privateupdateDisplay()
method to update theTextView
. - Now add the
onCreateDialog(int)
callback method to theHelloDatePicker
class:@Override protected Dialog onCreateDialog(int id) { switch (id) { case DATE_DIALOG_ID: return new DatePickerDialog(this, mDateSetListener, mYear, mMonth, mDay); } return null; }
This is an
Activity
callback method that is passed the integer ID given toshowDialog(int)
(which is called by the button'sView.OnClickListener
). When the ID matches the switch case defined here, aDatePickerDialog
is instantiated with theDatePickerDialog.OnDateSetListener
created in the previous step, along with the date variables to initialize the widget date. - Run the application.
When you press the "Change the date" button, you should see the following:
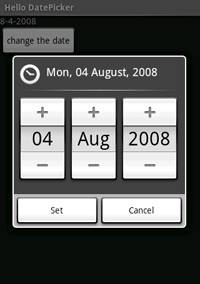